
Transitioning from React to React Native: Best for Web Developers 2024
React has become a go-to library for building dynamic web applications, offering developers powerful tools to create interactive UIs. With the growing demand for mobile apps, web developers often seek ways to leverage their skills to enter the mobile app domain. This is where transitioning from React to React Native becomes a game-changer, allowing web developers to create native mobile applications using familiar tools and concepts. This guide will cover the key aspects of making the switch from React to React Native and best practices to ease your journey.
Understanding the Differences between React and React Native
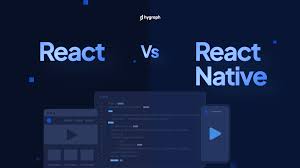
React is a JavaScript library used for building UIs, primarily for single-page applications (SPAs) and web development. It relies on HTML and CSS, paired with JavaScript, to render applications in the browser.
React Native, on the other hand, is a framework for building native mobile applications for iOS and Android. It allows developers to use JavaScript and React principles to build mobile applications that run natively on mobile devices.
Key differences:
- Rendering Mechanism: React uses HTML elements, while React Native uses native components specific to each platform.
- Styling: React relies on CSS for styling, whereas React Native uses a styling system similar to CSS but operates through JavaScript objects.
- Platform-specific Code: React is platform-agnostic (focused on browsers), while React Native has platform-specific capabilities to handle mobile requirements.
Why Transition from React to React Native?
Transitioning from React to React Native is beneficial for several reasons:
- Code Reusability: Leverage existing knowledge in React while creating mobile applications.
- Performance: Native components in React Native provide high performance, making it possible to build applications that feel like true native mobile apps.
- Wider Audience: React Native applications are cross-platform, meaning one codebase can serve both iOS and Android users.
- Time Efficiency: Reusing components and having a familiar structure significantly reduces development time.
For developers proficient in React, transitioning from React to React Native is a logical and highly rewarding step.
Step-by-Step Guide: Transitioning from React to React Native
1. Familiarize with Core Differences
To transition from React to React Native effectively, understanding key differences is essential. While React relies on the DOM (Document Object Model), React Native uses native UI components. For example, in React, you would use a <div>
element for containers, but in React Native, you would use <View>
.
React Native provides native equivalents of most HTML elements:
<View>
instead of<div>
<Text>
instead of<p>
or<span>
<Image>
instead of<img>
2. Setup Your React Native Environment
Before diving into code, set up a React Native development environment:
- Install Node.js and Watchman.
- Install React Native CLI or use Expo for easier setup and debugging.
- Choose a mobile emulator or connect a physical device for testing.
Having a stable environment helps when transitioning from React to React Native because you can see live updates as you make changes.
3. Relearning Styling in React Native
React developers are accustomed to CSS, but React Native handles styling a bit differently. Instead of external CSS files, you define styles as JavaScript objects within the component files:
javascriptCopy codeconst styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
});
This approach allows for styling similar to CSS, but with some changes. For instance:
- Use
flexDirection: 'column'
as the default rather thanrow
. - Text requires the
<Text>
component for styling, unlike in React where you can use<p>
,<h1>
, etc.
4. Managing State and Props
State and props work similarly in React and React Native, making the transition smoother. For instance, state can still be managed through the useState
hook, and complex state management can use useReducer
or libraries like Redux.
However, mobile applications often need additional state management due to the added complexities of mobile user interactions. Libraries such as React Navigation provide a structured way to handle navigation across different screens.
5. Handling Navigation in React Native
In web applications, navigation is typically handled through routers like React Router. When moving from React to React Native, you’ll encounter React Navigation, which is specially designed for mobile apps. This library provides various navigators such as Stack Navigator, Tab Navigator, and Drawer Navigator to help organize screens.
Example:
javascriptCopy codeimport { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator initialRouteName="Home">
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
6. Learning New Libraries for Mobile Functionality
Moving from React to React Native means you’ll need to understand libraries tailored for mobile functionality:
- Camera and Image Libraries: Libraries like
react-native-camera
andreact-native-image-picker
facilitate access to native mobile features. - Geolocation: Use
react-native-geolocation-service
to access device location. - Notifications: Libraries like
react-native-push-notifications
allow you to implement notifications within your app.
Each library is designed to bridge the gap between JavaScript code and native device functionality, providing a seamless experience for mobile users.
Common Challenges When Transitioning from React to React Native
- Platform Differences: iOS and Android handle components differently, so occasional platform-specific code is necessary. Use conditional rendering to differentiate between platforms when required.
- Device Constraints: Mobile devices have less computing power than desktops, so optimizing code for memory efficiency and smooth performance is critical.
- Learning Curve: Even though React and React Native are similar, the mobile environment requires additional knowledge about device hardware, native components, and user experience design for smaller screens.
Testing and Debugging in React Native
Testing is essential when moving from React to React Native as mobile applications require extensive testing on different devices and OS versions. Here are some tools to consider:
- Expo Go: Useful for real-time testing without needing to rebuild the app.
- React Native Debugger: This tool combines Redux DevTools and Chrome DevTools.
- Testing Libraries: Tools like Jest for unit testing and Detox for end-to-end testing ensure that your app functions as expected across scenarios.
Optimizing Performance in React Native
Performance optimization is a significant aspect when moving from React to React Native:
- Reduce Render Cycles: Optimize components to avoid unnecessary re-renders.
- Use Pure Components: Pure components or
React.memo
can help in minimizing render cycles for components with no state. - Optimize Images: Use
Image
component’s caching capabilities or load images in a lower resolution to save memory. - Manage Animations: Use libraries like
react-native-reanimated
for high-performance animations.
Best Practices for a Smooth Transition from React to React Native
- Keep Components Modular: Maintain reusable components to save development time.
- Optimize for Different Screens: Test responsiveness and layout adjustments to ensure your app looks great on various screen sizes.
- Code Reusability: Use shared libraries where possible to avoid redundant coding.
- Stay Updated: React Native evolves rapidly. Staying updated with the latest releases ensures you’re using the most efficient and stable code.
Conclusion: Is React to React Native Worth It?
Transitioning from React to React Native opens up opportunities to reach a vast audience through mobile platforms without starting from scratch. With the ability to use familiar React concepts, the learning curve is manageable, especially for experienced React developers. By leveraging the power of React Native, you can create powerful, high-performance mobile applications that meet the demands of modern users.
This step-by-step guide equips you to make the transition from React to React Native with ease. As more users rely on mobile experiences, moving from React to React Native not only expands your development capabilities but also broadens your career prospects in mobile app development.